In Python, working with files is an essential skill that allows developers to store and retrieve data. One of the most important concepts related to files is mode files. These are specific settings that determine how a file is opened and how the program interacts with it. In this article, we will explore mode files in Python, their different types, and how to use them effectively in your Python projects. Understanding file modes will help you read, write, and manipulate data seamlessly, making your programs more dynamic and efficient.
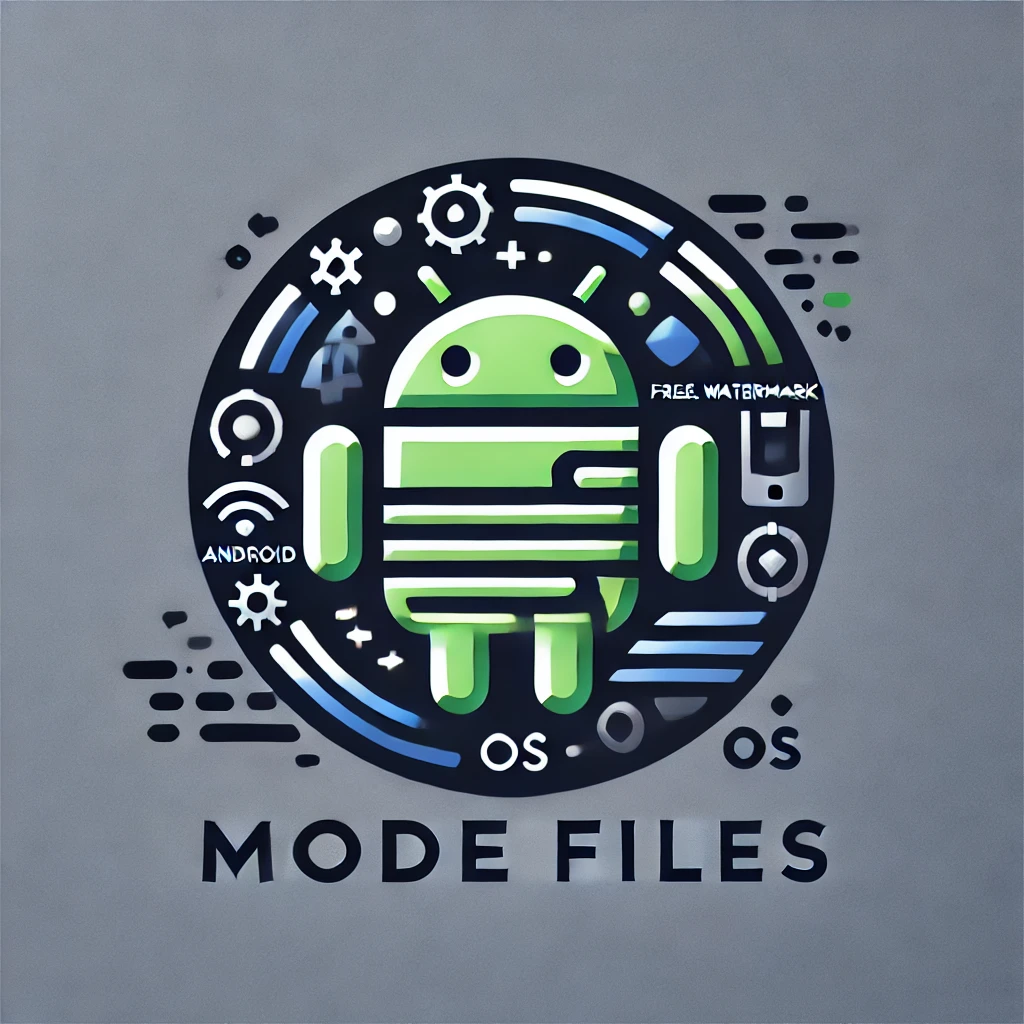
What Are Mode Files?
In Python, mode files are parameters that define the actions allowed on a file. They specify whether a file should be opened for reading, writing, or appending. These modes also control whether the file is opened in binary or text format. When you open a file using the built-in open()
function in Python, you must specify the mode in which you want the file to be opened.
Each mode serves a specific purpose and allows the program to interact with the file in different ways. Understanding how these modes work is essential for managing file operations efficiently.
Common Modes for Opening Files in Python
There are several modes available when working with files in Python. Below are the most commonly used modes:
1. Read Mode ('r'
)
The 'r'
mode is used when you want to read a file. When a file is opened in this mode, Python will open the file for reading only. If the file does not exist, it will raise a FileNotFoundError
. This is the default mode if no mode is specified.
Example:
pythonCopy codefile = open("example.txt", "r")
content = file.read()
print(content)
file.close()
In this example, the file is opened for reading, and the content is printed.
2. Write Mode ('w'
)
The 'w'
mode is used when you want to write to a file. If the file already exists, its content will be erased, and the new data will be written. If the file does not exist, it will be created.
Example:
pythonCopy codefile = open("example.txt", "w")
file.write("Hello, world!")
file.close()
This example will create (or overwrite) the file example.txt
and write „Hello, world!“ to it.
3. Append Mode ('a'
)
The 'a'
mode allows you to append data to an existing file. If the file does not exist, it will be created. Unlike the 'w'
mode, the 'a'
mode does not overwrite existing content but adds new data at the end of the file.
Example:
pythonCopy codefile = open("example.txt", "a")
file.write("Adding new content!")
file.close()
This will add „Adding new content!“ to the end of the file example.txt
.
4. Read-Write Mode ('r+'
)
The 'r+'
mode allows you to read and write to a file simultaneously. The file must already exist for this mode to work. If the file is not found, it will raise an error. This mode is useful when you need to modify specific parts of a file without deleting its content.
Example:
pythonCopy codefile = open("example.txt", "r+")
content = file.read()
file.seek(0)
file.write("Modified content.")
file.close()
This example reads the content of the file and then writes „Modified content“ at the beginning of the file.
5. Binary Read Mode ('rb'
)
The 'rb'
mode opens a file for reading in binary format. This is useful when you are working with non-text files like images, videos, or audio files.
Example:
pythonCopy codefile = open("example.jpg", "rb")
content = file.read()
file.close()
In this example, the file is opened in binary mode to read its contents.
6. Binary Write Mode ('wb'
)
The 'wb'
mode allows you to write in binary format. It is used when you need to write non-text data to a file, such as images or files containing special characters.
Example:
pythonCopy codefile = open("example.jpg", "wb")
file.write(image_data)
file.close()
Here, the image_data
is written to the file in binary format.
7. Binary Append Mode ('ab'
)
The 'ab'
mode is used to append binary data to an existing file. Like the 'a'
mode for text, this mode adds data to the end of a binary file.
Example:
pythonCopy codefile = open("example.jpg", "ab")
file.write(new_image_data)
file.close()
This example appends new_image_data
to the existing binary file.
Using Mode Files in Python: Practical Examples
Let’s look at some practical examples to understand how mode files in Python can be used in real-world programming.
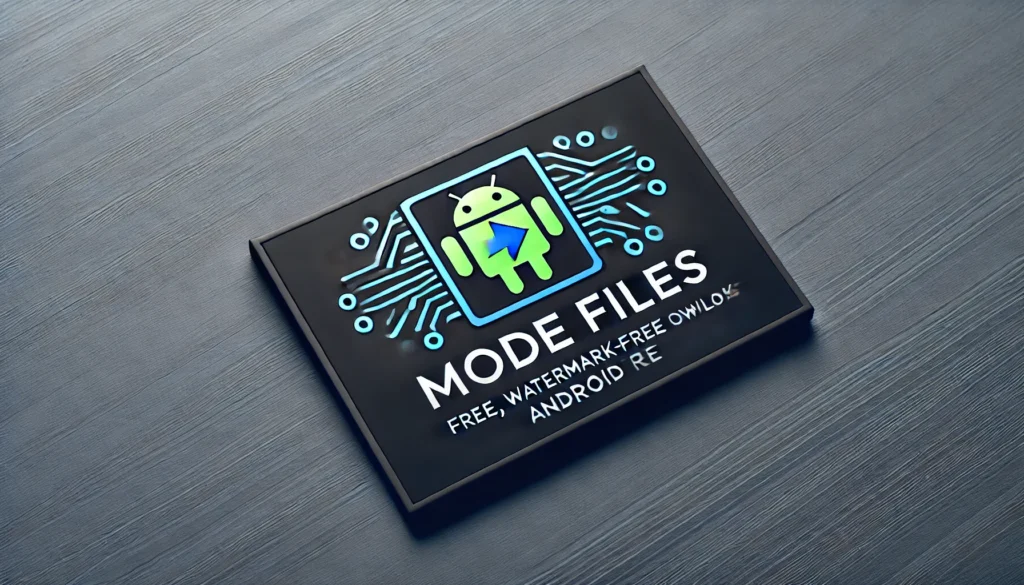
Example 1: Reading from a Text File
In this example, we’ll open a text file in read mode and print its content.
pythonCopy codefile = open("data.txt", "r")
data = file.read()
print(data)
file.close()
Here, the program opens the file data.txt
, reads the content, and prints it.
Example 2: Writing to a File
Let’s see how to write content to a file in write mode.
pythonCopy codefile = open("output.txt", "w")
file.write("This is a new line of text.")
file.close()
This will create output.txt
(or overwrite it if it already exists) and write the specified text to it.
Example 3: Appending Data to a File
Now, we’ll append some text to an existing file using append mode.
pythonCopy codefile = open("output.txt", "a")
file.write("\nAppended text.")
file.close()
This code will add a new line with the text „Appended text.“ to the file output.txt
.
Working with File Modes in Python: Best Practices
When working with mode files in Python, consider the following best practices:
- Always Close Files: After performing any file operation, it’s important to close the file to save changes and free up system resources. This can be done using the
file.close()
method. - Use
with
Statement: Instead of manually opening and closing files, you can use thewith
statement, which automatically takes care of closing the file after operations are done.Example:pythonCopy codewith open("output.txt", "w") as file: file.write("This will be automatically saved.")
- Error Handling: Use try-except blocks to handle errors like file not found or permission issues when working with files.Example:pythonCopy code
try: file = open("nonexistent.txt", "r") except FileNotFoundError: print("The file does not exist.")
- Check File Existence: Before performing write operations, it’s a good practice to check if the file already exists, especially if you don’t want to overwrite important data.
Conclusion
Understanding mode files in Python is crucial for managing file operations effectively. The different file modes allow you to control how data is read, written, or appended in files. By using the correct mode for your task, you can ensure that your program works efficiently and handles data in the best possible way. Whether you are dealing with text files or binary files, knowing when and how to use each mode will help you manage files seamlessly in your Python projects.
For more…… Click here.